When working with C++ templates, you may encounter the error “use of class template requires template argument list” which indicates that a template class is being used without specifying the necessary template arguments. Understanding the purpose and resolution of this error is crucial for efficient template programming. This article aims to provide a comprehensive explanation of this error and guide you through the proper usage of class templates.
Templates are a powerful feature in C++ that allow you to create generic code that can work with different data types or objects. Class templates are used to create generic classes that can be instantiated with different types. To use a class template, you need to provide the appropriate template arguments when instantiating the class. Failure to provide the template arguments results in the “use of class template requires template argument list” error.
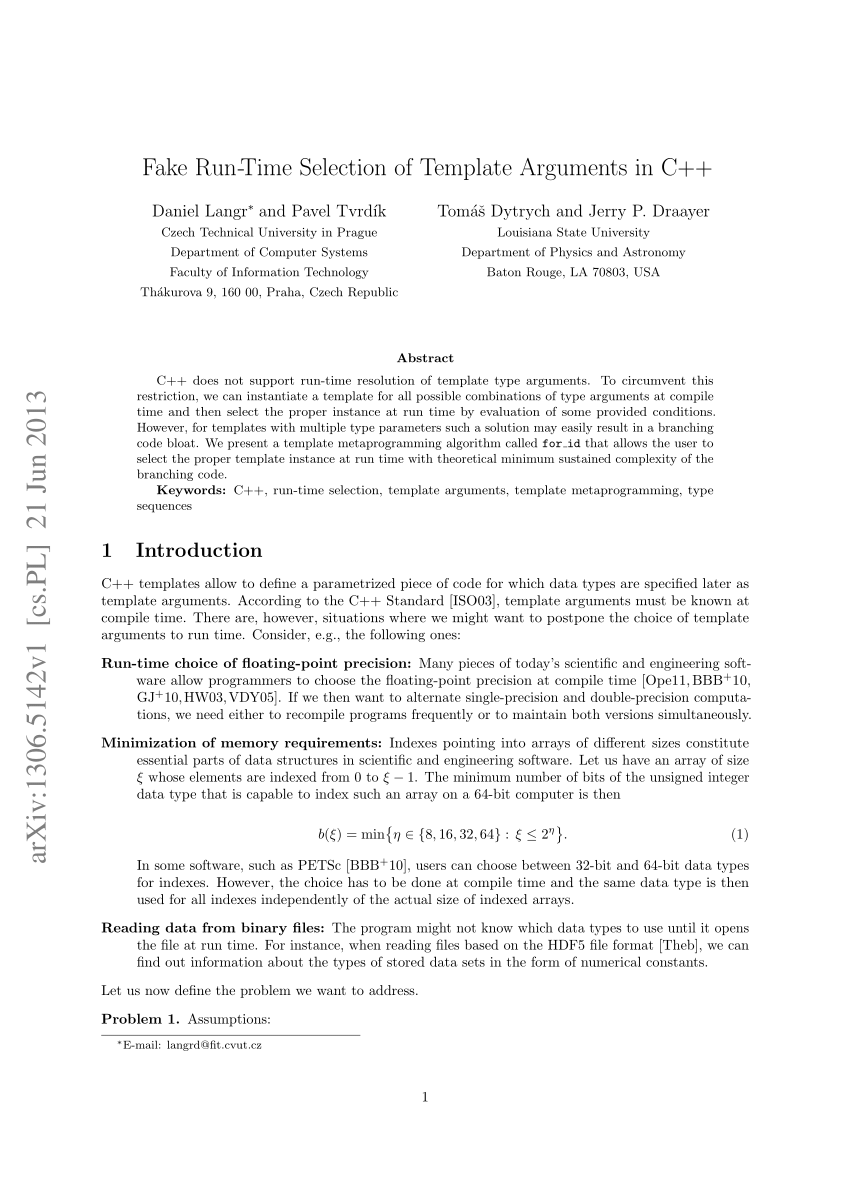
Understanding the Error
The “use of class template requires template argument list” error occurs when you try to use a class template without specifying the template arguments. This error message indicates that the compiler needs additional information to create a specific instance of the class template. Without the template arguments, the compiler cannot determine the type of data or objects that the class template will work with.
To resolve this error, you need to provide the necessary template arguments when instantiating the class template. The template arguments specify the types or objects that the class will work with. By providing the template arguments, you instruct the compiler on how to create a specific instance of the class template.
Proper Usage of Class Templates
To correctly use class templates, follow these steps:
- Declare the class template with template parameters as placeholders for the types or objects it will work with.
- When instantiating the class template, provide the actual types or objects as template arguments within angle brackets (<>) after the class name.
For example, if you have a class template called `MyClass` that takes a single template parameter `T`, you would instantiate it as follows:
“`cpp
MyClass
MyClass
“`
By providing the template arguments, the compiler can create specific instances of the `MyClass` template for `int` and `std::string`. These instances can then be used to work with the respective types.
Conclusion
The “use of class template requires template argument list” error occurs when a class template is used without specifying the necessary template arguments. To resolve this error, you need to provide the appropriate template arguments when instantiating the class template. Understanding the purpose and resolution of this error is essential for efficient template programming. By following the proper usage guidelines, you can effectively utilize class templates to create generic and reusable code.
Remember, when working with class templates, always ensure that you provide the necessary template arguments to avoid the “use of class template requires template argument list” error. This ensures that the compiler can create specific instances of the class template that meet your requirements.