In C++, templates are used to create generic code that can be used with different data types. Class templates are templates that define classes, and can be used to create objects of that class with different data types. However, when using class templates, it’s important to understand the concept of template argument lists.
A template argument list specifies the types or values that are used to instantiate the template. For class templates, the template argument list must be provided when the class is instantiated, and it specifies the data type of the objects that will be created from the class. Failure to provide a template argument list when instantiating a class template will result in a compiler error.
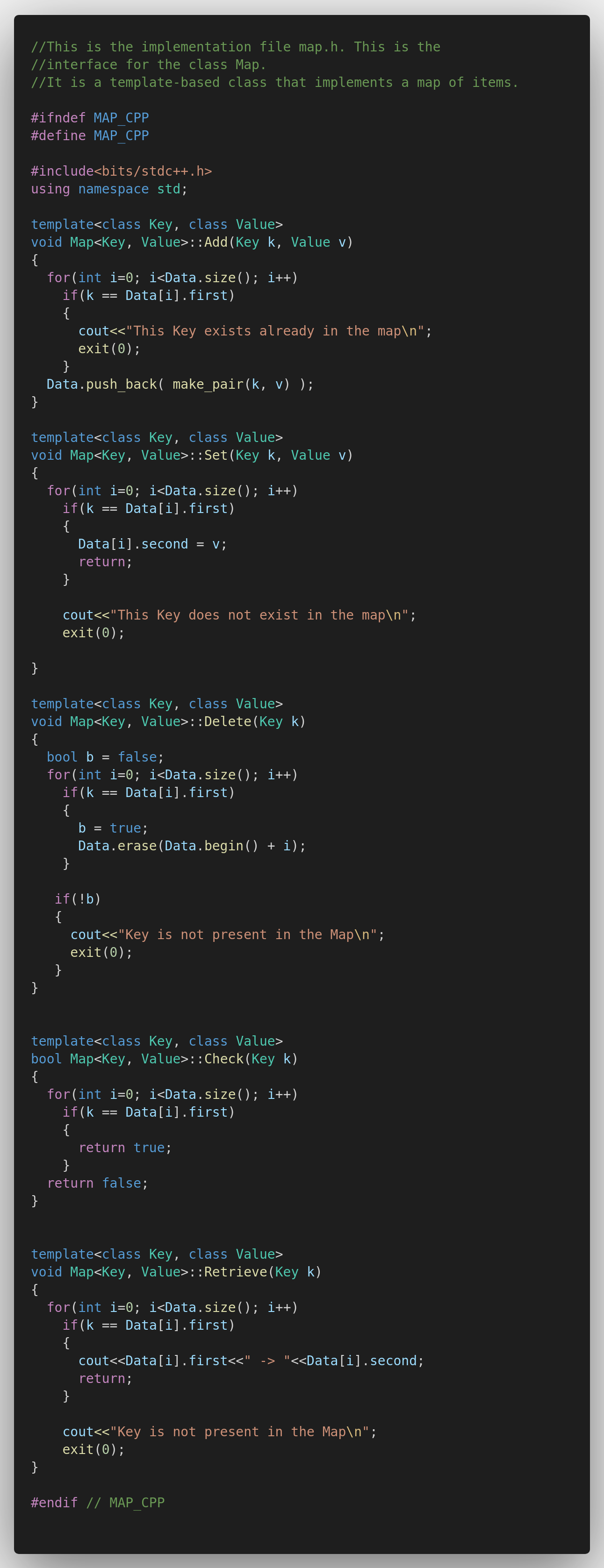
Understanding Template Argument Lists
Template argument lists are enclosed in angle brackets (<>) and follow the class template name. The arguments in the list can be types, constants, or expressions. For example, the following code defines a class template called Array
that can be used to create arrays of any data type:
“`c++
template
class Array {
T* data;
int size;
};
“`
To instantiate the Array
class template, you must provide a template argument list. For example, the following code creates an array of integers:
“`c++
Array
“`
In this example, the template argument list is Array
class will be instantiated with the int
data type. This means that the myArray
object will be an array of integers.
Default Template Arguments
In some cases, it may be desirable to provide default values for template arguments. This can be done by specifying the default value after the argument name in the template parameter list. For example, the following code defines a class template called Vector
that has a default template argument of int
:
```c++
template
class Vector {
T* data;
int size;
};
```
If no template argument is provided when instantiating the Vector
class, the default template argument of int
will be used. For example, the following code creates a vector of integers:
```c++
Vector<> myVector;
```
Conclusion
Template argument lists are an essential part of using class templates in C++. They allow you to specify the data types or values that will be used to instantiate the template, and can help to make your code more generic and reusable.
By understanding how to use template argument lists, you can take full advantage of the power of C++ templates to create efficient, flexible, and maintainable code.